JavaScript plays a pivotal role in developing websites. Around 97% of the websites have adopted the tool for its web page behavior. It is a dynamic programming language that you can use for various purposes like web development, in web application, game development, and more. You can even implement dynamic features on your web pages to enhance their look that cannot be achieved with HTML and CSS. JavaScript is a single-threaded language that has one call stack and one memory heap. Here single thread is the main browser thread. However, it can be non-blocking while using asynchronous executions. JavaScript handles asynchronous executions using the call stack, callback queue, web API, and event loop which are unrelated to workers.
What are JavaScript workers?
In simple terms, JavaScript workers run on a thread other than the main browser thread. So, when we execute these scripts, they do not block the main line and the browser remains responsive. In this blog, I aim to cover web workers and service workers. I have explained both these types of workers and how they are used along with their purpose.
Web Workers
While executing JavaScript in the browser, it uses the browser main (single) thread. This blocks the other execution until the current script’s execution is finished. Thus, leaving the browser unresponsive until it finishes executing the existing script. Web Workers, on the other hand, runs independently and does not affect the browser page performance. Web workers are supported in almost all known browsers, and this can be used to do some expensive calculations without leaving the browser unresponsive.
Web Workers Creation
Web workers are simple JavaScript files that can run by creating new workers object using the workers file. A simple workers file can look like,
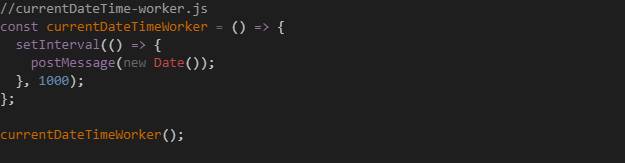
Workers can be instantiated like,

Communication With Workers
The Communication process between workers and client will be achieved by listening to messages from each other. Below code is a sample to add a listener for both client and workers in which they have implemented onmessage function, which is the listener. To send messages to the workers and clients, use the postMessage process to post messages to each other.


Terminating Workers
Service workers instance has terminate function that can be called to terminate.

Service Workers
Service workers are specialized workers that run in the background and are separate from a web page. This acts like a proxy network and cache that helps developers build an offline experience for the web pages. Like web workers service, worker also uses postMessage API to communicate from client web pages. Since this can act as a proxy, the registration of service workers is restricted to pages served over HTTPS. For development purposes, localhost can be used to register the service workers.
Let’s discuss the service workers lifecycle and communication with the client. Here, I have taken an example of a web page with some static content and some dynamic forms in which users can upload data.
Service Workers Registration
To register service workers, a web page should provide a path to the service workers file as given below:
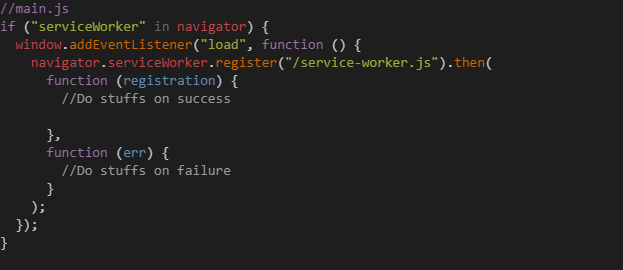
Here, the service workers are placed at the root path. This way, the service workers can intercept fetch events in your domain. If the service workers are registered with path /example/service-worker.js then the service workers can intercept all the fetch events starts with /example/. Eg. /example/abc or /example/xyz.
Service Workers Installation
When a page tries to register service workers, it kicks off the install listener that is present in the service workers file. Through this event the listener is triggered when the service workers are first installed. The installed event can look like as mentioned below:
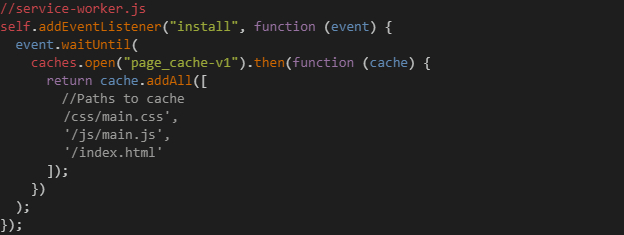
In the above example files, main.css, main.js and index.html will be added to the cache.
Service Workers Activation
When the service workers are installed, it enters into the next phase which is activation. We can clean the old cache during the activation and set up for IndexedDB to store some dynamic data. In the example we have a form in which there are some text fields and some files to be uploaded. We can set up IndexedDB to store those files in case the user goes offline while saving the form data to the server.
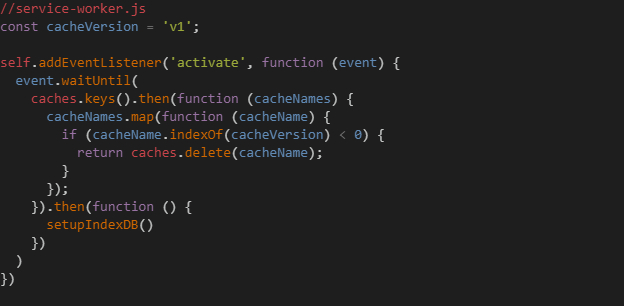
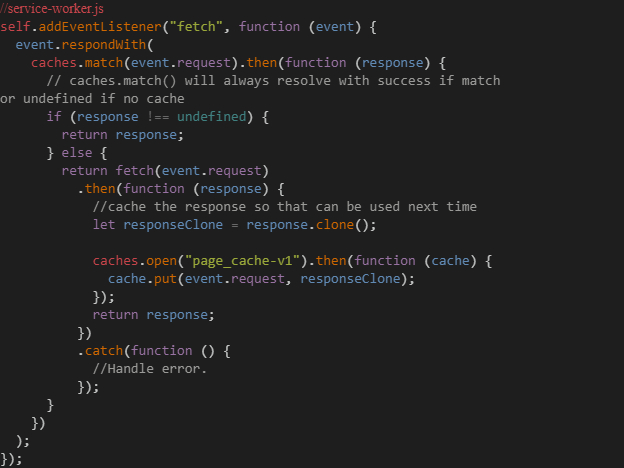
Communication With Service Workers
The communication between the client and service workers happens through postMessage API. There are several ways a client can communicate through service workers. Let’s take the above example where the user has made some entries in the form and failed to upload it because of the unavailability of the network.
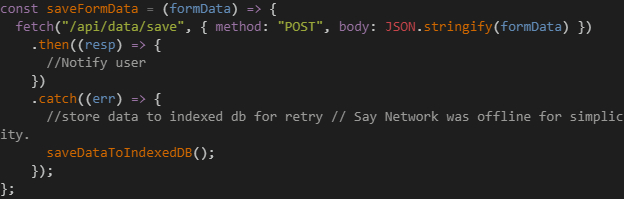
Here, we will be discussing the client page that is making a request to the service workers to upload and show the communication from the service workers. Client pages have a listener to the online event. When the network is online, the client requests the service workers to retry the upload. When the network connection is restored, try uploading the form data to the server by communicating with the service workers on-demand, or the service workers make it automatically by checking the status of upload items present in IndexedDB.
- Using Broadcast Channel
As one can guess, the Broadcast channel allows us to communicate to browsing contexts. In this case, both the client and the service workers use the same track to communicate. It is the most effortless setup for communication; however, not all browsers currently support the broadcast channel.
Note: Check the browser support before using it.
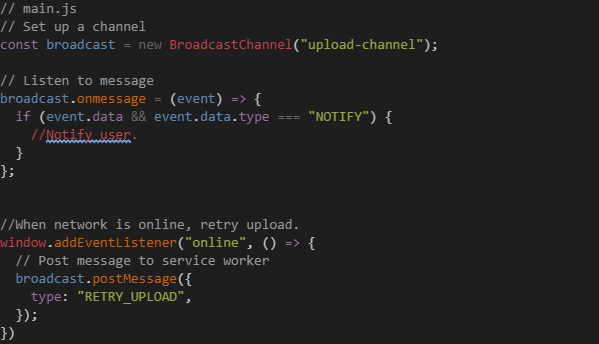
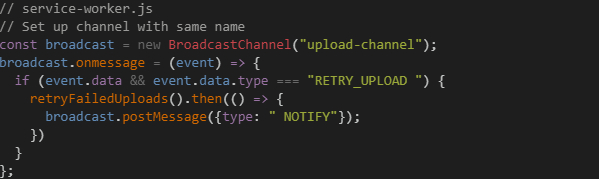
Let’s assume retryFailedUpload function has a job that is to retrieve data from IndededDB and then make a network request to save the data to return a promise indicating success/failure.
2. Using Client API
The service workers have all the clients and can communicate through one more client by sending messages to more clients. Service workers have references to connected clients ordered by the last focused client. The service workers can target clients and post a message. Thus, the client can use the service workers controller to send messages to the service workers.
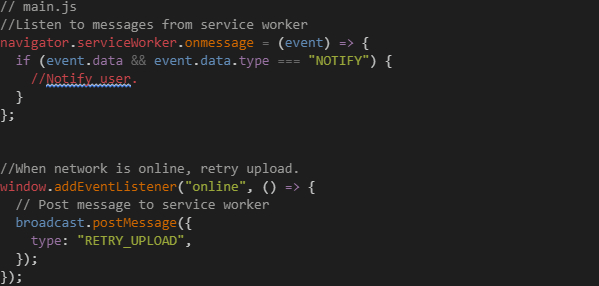

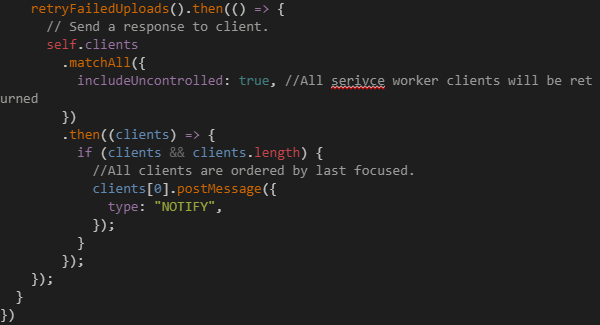
3. Using Channel Messaging
Using channel messaging, we can listen to the messages for a channel port sent by the service workers Message channel instance contains two ports, namely port1 and port2. The client can listen to messages from the service workers and the service workers send the messages to the listening port. In the code below, the first client sends a message to keep a reference of the client port on which the service workers can send the messages.
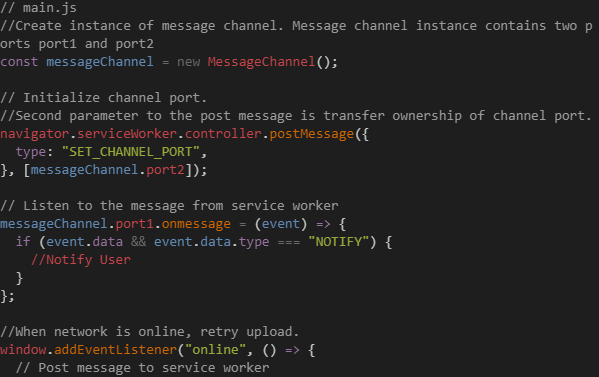
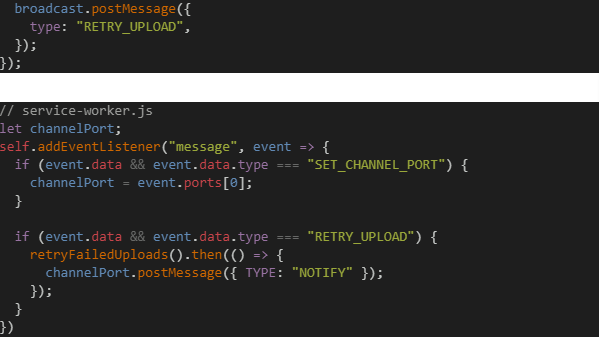
Uses and More
Web workers are helpful when performing long-running tasks or some periodic tasks. It does not have a restriction on the number of workers created. A page can have multiple web workers; also, a worker can instantiate workers.
On the other hand, the service workers are meant for boosting the offline experience. So far, we have discussed mainly caching, but service workers can do periodic sync and manage notifications. I hope this brief overview of the JavaScript workers help you to understand it better. Till then, happy coding!